How to round up in VBA, VB6 and VB.net: rounded, Int
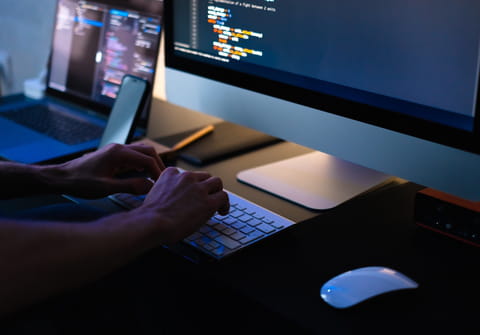
The Round() function returns a number rounded to the specified number of decimal places (2, down...). However, sometimes this function does not perform the operation correctly. In this article we show you how to solve it using another method.
How to use the Round() function?
The Round() function consists of two parts: the numeric expression you want to round and the number of decimal places. Below we show an example of an incorrect operation:
Round(0.15 , 1) = 0.2 Round(0.25 , 1) = 0.2 Round(0.35 , 1) = 0.4 Round(0.45 , 1) = 0.4
What is an alternative to the Round() function?
The following function, called Rounded(), does not cause this problem:
Private Function Rounded(ByVal Number, ByVal Decimals) Rounded = Int(Number * 10 ^ Decimals + 1 / 2) / 10 ^ Decimals End Function
It gives us the following result:
Rounded(0.15 , 1) = 0.2 Rounded(0.25 , 1) = 0.3 Rounded(0.35 , 1) = 0.4 Rounded(0.45 , 1) = 0.5
How to round to 2 decimal places in VBA?
To round to 2 decimal places in VBA, you can use the Round function as follows:
numberRounded = Round(number, 2)
As we mentioned before, this function allows you to round a number to the specified number of decimal places, in this case, 2 decimal places. It is very useful when you need to display values with a certain precision, such as in financial or scientific calculations.
How to round down in VBA?
To round down in VBA, you can use the Int function as follows:
numberRounded = Int(number)
The Int function rounds a number to an integer. This is useful when you need to eliminate decimals and work only with whole numbers.